You’ve recently taken a job designing games for casinos. You’ve been assigned to build a game simulating an 8-horse race. In the game, you play against the house, and some number of AI NPCs. The more quarters you pump into the machine, the more Player horses there are, and the fewer NPCs there are, increasing your chances of winning. A mockup of what the game might look like is below, but feel free to be more creative!
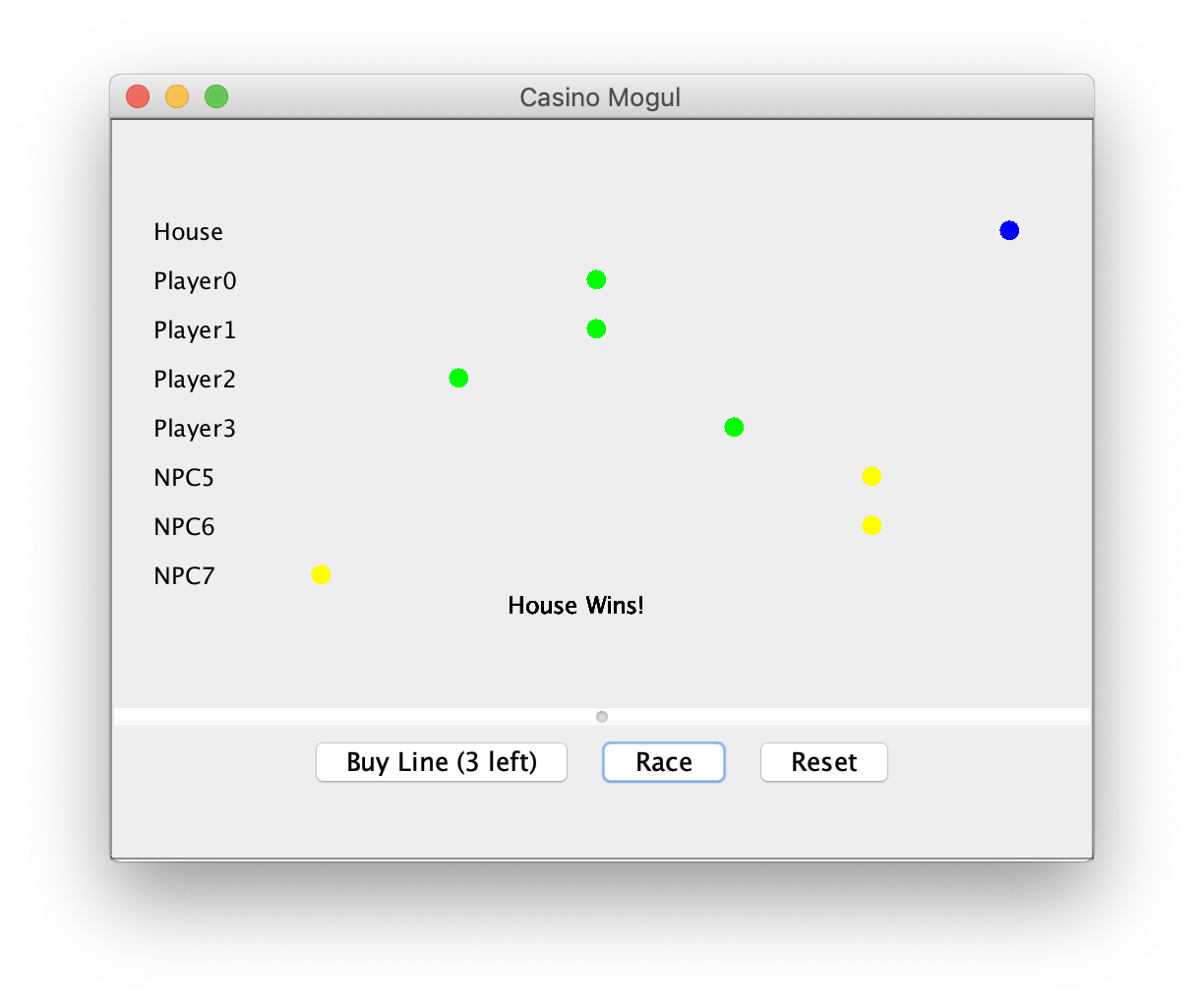
Of course, like all good casino games, the game is rigged in favor of the house. The player and each NPC each have a 10% chance of being faster than the house’s horse. Of course, the house wins all of the money whenever an NPC wins, too. Specifically, you will do the following:
- Create a class hierarchy containing the Horse class, the Player class, the NPC class, and the House class. The Horse class will never be instantiated on its own. Each horse has:
- an identifier, based on its type (e.g., House, Player1, Player2, …, NPC1, NPC2, …);
- a color, based on its type (e.g., red for the house, blue for the player, and yellow for the NPC);
- a speed the horse moves. The house’s horse moves at a speed of 1.0, while each the NPC and player horse has a 10% probability of having a speed greater than that of the house. At instantiation, the speed should be generated randomly for NPC and Player horses, according to the probability of a win;
- a current location;
- a method for painting itself. Each horse is represented as a dot, along with the identifier for the horse. The dot should be the color specified in the appropriate horse class. The dot’s position along the X axis represents the current location of the horse;
- a method for advancing the horse an amount equal to 5 times the speed. This method should take as an argument a callback method for reporting that the horse has won.
- any other methods you think might be useful
- Create a class called HorseRace which contains the state of the race itself. It contains:
- An array of 8 horses (one for each lane);
- A method for advancing all of the horses one step;
- A method for getting the winner, if there is one;
- A method for painting all of the horses;
- Possibly a method for resetting the race back to its initial condition (depending on your design choices);
- Any other methods you think might be useful.
- Create a class called CasinoMogulApp which (perhaps using one or more JPanels):
- Manages the HorseRace object;
- Contains a region where the horses are painted;
- Contains three buttons: Buy Line, Race, and Reset;
- When the user presses the Buy Line button, it should (somehow) swap out one of the horses from an NPC to a Player;
- When the user presses the Race button, the race should commence. The race is over when one horse has traveled 400 units. The identifier for the winning horse should be displayed. You should use a Swing Timer as you did in the previous project to animate the race progress;
- When the user presses the reset button, the race should return to its initial state (with the Player having no lines).
This project is designed to examine your skill at using object oriented principles (encapsulation, inheritance, polymorphism). You should be sure you are using these appropriately!